With the help of C programming, we can easily solve various mathematical problems like division, multiplication, etc. In this tutorial, we are going to write a C program to find the average of numbers.
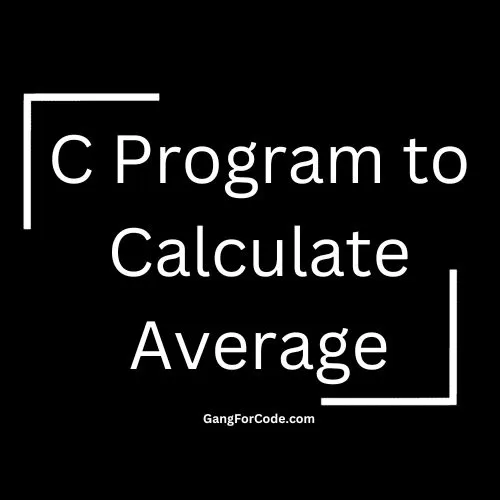
Table of Contents
How to Calculate Average in C Programming
The logic for calculating the average of numbers is simple. We will ask users to enter the count of the numbers they want to average after that we ask the users to enter each number. After that, we will calculate the sum of the numbers and divide the sum by the count of the numbers.
C Program to Calculate Average
Let’s write a C program to calculate the average of given numbers.
#include <stdio.h>
void main()
{
int count, i;
float number, average, sum = 0;
printf("Enter the count of numbers ");
scanf("%d", &count);
for (int i = 1; i <= count; i++)
{
printf("Enter %d number ", i);
scanf("%f", &number);
sum = sum + number;
}
average = sum / count;
printf("Average of given numbers = %f", average);
}
Output

This is a simple approach to calculating the average of n numbers using a C program, by using the same logic we can also calculate the average of n numbers in other programming languages like C++, Java, Python, Rust, etc.
Happy Coding
2 thoughts on “C Program to Calculate Average”