Unit testing is a vital part of software development that ensures individual components of your application work as intended. In Java, the most popular framework for unit testing is JUnit, currently in its fifth iteration (JUnit 5). This guide provides an in-depth explanation of Java unit testing and demonstrates its implementation with practical examples.
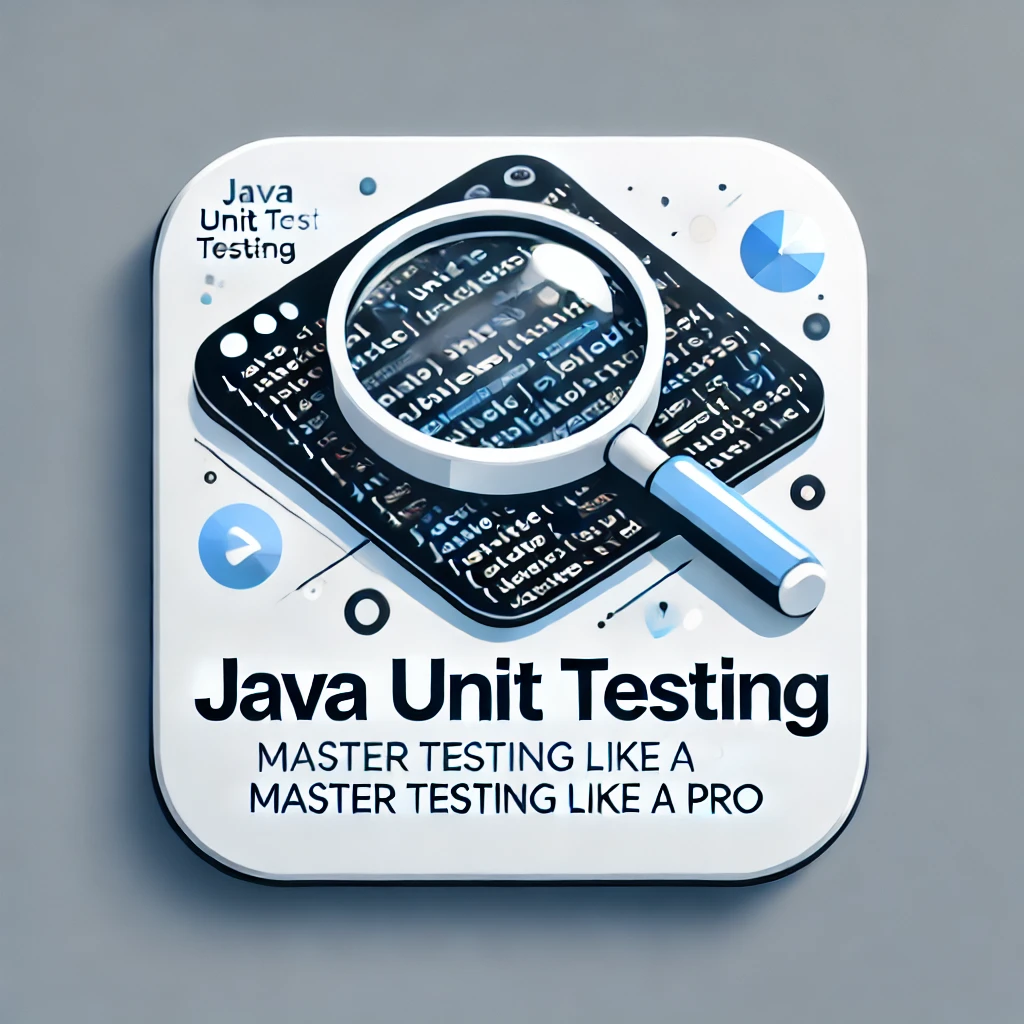
Table of Contents
What is Unit Testing?
Unit testing is the process of verifying the smallest testable parts (units) of a program, such as methods or functions, to ensure they behave correctly. It isolates these units to validate their logic without interference from external dependencies.
Benefits of Unit Testing
- Code Reliability: Identifies and fixes bugs early in development.
- Refactoring Confidence: Ensures existing functionality isn’t broken by code changes.
- Documentation: Tests act as examples for understanding code behavior.
- Faster Debugging: Pinpoints problems in specific units.
Setting Up JUnit for Java
JUnit is a widely used testing framework in Java. To begin:
Add JUnit Dependency
For Maven
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter</artifactId>
<version>5.9.3</version>
<scope>test</scope>
</dependency>
For Gradle
gradle dependencies { testImplementation 'org.junit.jupiter:junit-jupiter:5.9.3' }
Set Up Your IDE: Most IDEs like IntelliJ IDEA and Eclipse natively support JUnit integration.
Key Concepts of Unit Testing
Annotations in JUnit
JUnit provides annotations to define and organize your test cases:
@Test
: Marks a method as a test method.@BeforeEach
: Runs before each test method.@AfterEach
: Runs after each test method.@BeforeAll
: Runs once before all tests (static method).@AfterAll
: Runs once after all tests (static method).@Disabled
: Disables a test method or class.
Assertions
JUnit provides methods to verify the expected results:
assertEquals(expected, actual)
assertTrue(condition)
assertFalse(condition)
assertNull(value)
assertNotNull(value)
Example Implementation
Here’s a practical example of using JUnit to test a simple calculator class.
Step 1: Create the Class to Test
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public int subtract(int a, int b) {
return a - b;
}
public int multiply(int a, int b) {
return a * b;
}
public int divide(int a, int b) {
if (b == 0) {
throw new IllegalArgumentException("Division by zero is not allowed");
}
return a / b;
}
}
Step 2: Write Unit Tests
Create a test class, typically named CalculatorTest
.
import org.junit.jupiter.api.*;
import static org.junit.jupiter.api.Assertions.*;
class CalculatorTest {
private Calculator calculator;
@BeforeEach
void setUp() {
calculator = new Calculator();
}
@Test
void testAdd() {
assertEquals(5, calculator.add(2, 3));
assertEquals(0, calculator.add(-1, 1));
}
@Test
void testSubtract() {
assertEquals(1, calculator.subtract(3, 2));
assertEquals(-2, calculator.subtract(-1, 1));
}
@Test
void testMultiply() {
assertEquals(6, calculator.multiply(2, 3));
assertEquals(0, calculator.multiply(0, 5));
}
@Test
void testDivide() {
assertEquals(2, calculator.divide(4, 2));
assertThrows(IllegalArgumentException.class, () -> calculator.divide(1, 0));
}
}
Output
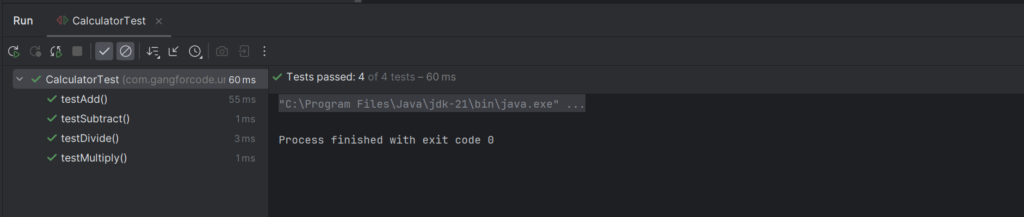
Running the Tests
- In your IDE, right-click on the test class or method and select “Run”.
- If using the command line:
- With Maven:
mvn test
- With Gradle:
gradle test
Successful tests will be marked as PASSED, while failures provide details on discrepancies.
Advanced Features
Mocking Dependencies
For testing methods that rely on external services, you can use mocking libraries like Mockito.
import static org.mockito.Mockito.*;
public class ServiceTest {
@Test
void testServiceMethod() {
ExternalService mockService = mock(ExternalService.class);
when(mockService.getData()).thenReturn("Mock Data");
Service service = new Service(mockService);
assertEquals("Mock Data", service.fetchData());
}
}
Parameterized Tests
JUnit 5 allows tests to run multiple times with different parameters.
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.ValueSource;
import static org.junit.jupiter.api.Assertions.*;
class ParameterizedTestExample {
@ParameterizedTest
@ValueSource(ints = {1, 2, 3, 4})
void testEvenNumbers(int number) {
assertTrue(number % 2 == 0);
}
}
Best Practices for Unit Testing
- Test One Thing at a Time: Each test should validate a single functionality.
- Name Tests Clearly: Use descriptive names like
testAddWithPositiveNumbers
. - Isolate Tests: Avoid dependencies between test cases.
- Mock External Dependencies: Use mocking to isolate units from integrations.
- Maintain Test Coverage: Ensure all critical paths and edge cases are tested.
Conclusion
Unit testing in Java with JUnit helps create robust, maintainable, and bug-free code. By following the steps and examples provided, you can integrate unit testing into your workflow effectively. Start small, test often, and make testing an integral part of your development process.