As a powerful tool in the field of Java and enterprise application dynamism, Spring Boot simplifies initial project setup, reduces development time, and effortlessly manages dependencies with a powerful annotation-based configuration. This article serves as a comprehensive cheat sheet on the numerous annotations available in Spring Boot, promising to provide simple, ready-to-use tips for developers.
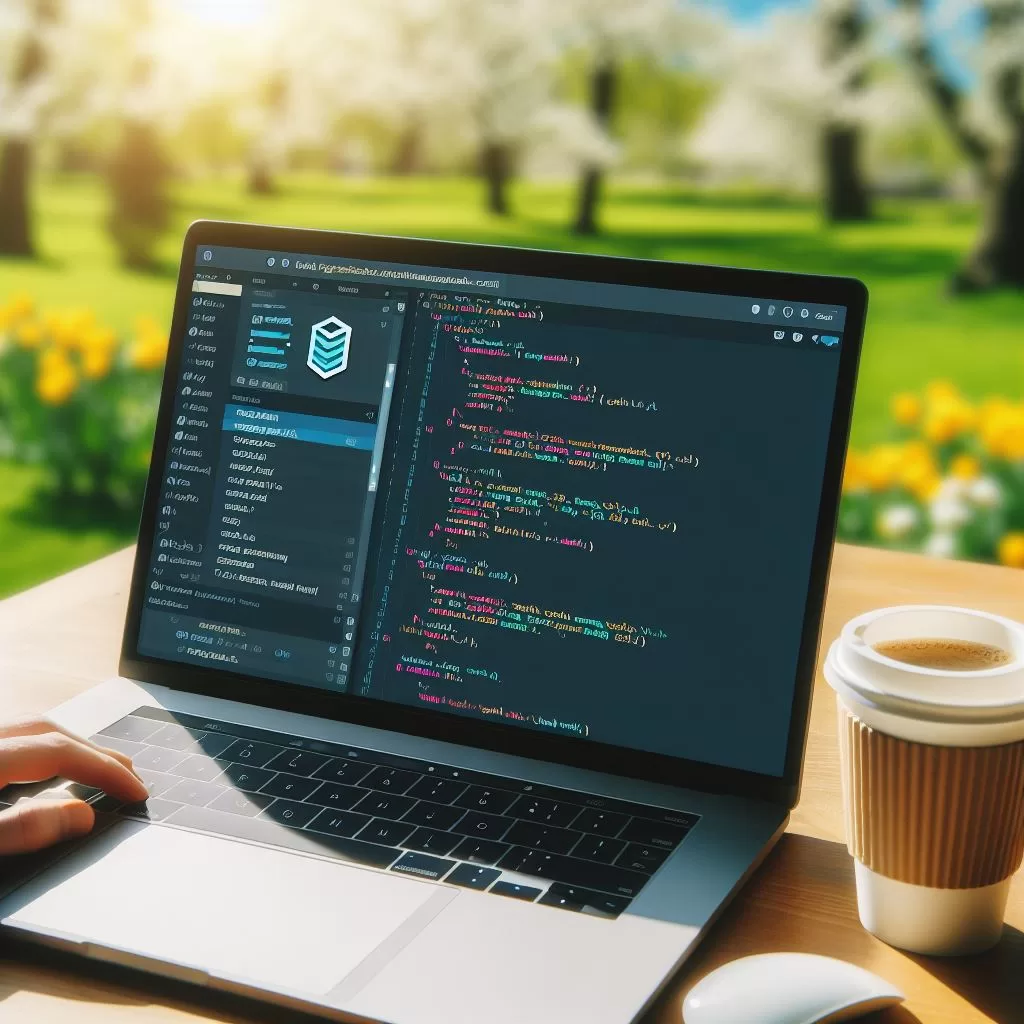
Table of Contents
Spring Boot Annotations Cheat Sheet
Core Annotations
- @SpringBootApplication: This meta-annotation combines @Configuration, @EnableAutoConfiguration, and @ComponentScan, marking the main class as your application’s entry point.
- @Configuration: Marks a class as a source of bean definitions.
- @EnableAutoConfiguration: Enables Spring Boot to automatically configure beans based on the dependencies present on the classpath.
- @ComponentScan: Specifies base packages to scan for annotated components (@Component, @Service, @Repository).
Bean Management Annotations
- @Component: Marks a class as a Spring bean managed by the container.
- @Service: Specialization of @Component for service-layer beans.
- @Repository: Specialization of @Component for repository-layer beans.
- @Controller: Marks a class as a web controller handling HTTP requests.
- @Bean: Explicitly defines a bean within a configuration class.
- @Autowired: Injects dependencies into other beans.
- @Qualifier: Differentiates between multiple beans of the same type.
- @Scope: Defines the scope of a bean (singleton, prototype, etc.).
Web Annotations
- @RequestMapping: Maps a controller method to a specific URL or HTTP method.
- @GetMapping: Shortcut for @RequestMapping(method = RequestMethod.GET).
- @PostMapping: Shortcut for @RequestMapping(method = RequestMethod.POST).
- @RequestParam: Maps a web request parameter to a method parameter.
- @PathVariable: Extracts path variables from the URL and maps them to method parameters.
- @RequestBody: Deserializes the HTTP request body into a Java object.
- @ResponseBody: Serializes the method return value into the HTTP response body.
Other Useful Annotations
- @Conditional: Configures beans based on specific conditions.
- @Profile: Specifies different configurations for different environments (e.g., dev, prod).
- @PropertySource: Defines external properties files to be loaded.
- @Value: Injects property values from external sources (e.g., properties files, environment variables).
- @ConfigurationProperties: Binds properties from external sources to an annotated POJO class.
Spring Boot Annotations Detailed Explanation
@SpringBootApplication
The cardinal annotation of the Spring Boot framework, @SpringBootApplication, combines three noteworthy annotations in one – namely @Configuration
, @ComponentScan
, and @EnableAutoConfiguration
. Typically, it is located at the primary class of your application, and it identifies packages to scan for Beans, additional configurations, and application contexts.
@EnableAutoConfiguration
As a part of the @SpringBootApplication, @EnableAutoConfiguration dutifully attempts to guess and configure the beans that you will likely need. Essentially, it is letting Spring Boot to make intelligent, educated decisions on your behalf.
@ComponentScan
Another integral aspect of the @SpringBootApplication is the @ComponentScan. This annotation commands Spring to look for other components, configurations, and services within the pre-defined package, thereby facilitating scanning for configurations.
@Configuration
Working in sync with @ComponentScan, @Configuration allows methods annotated with @Bean to be automatically included in the Spring’s Context, thus providing functionality to configure Spring Containers by turning classes into @Beans.
@Bean
A necessary tool for manually defining a bean, @Bean allows Spring Boot to use a method as the source of a bean when auto-configuration isn’t the top priority. The @Bean annotation works in conjunction with Java configuration defined by @Configuration.
@Autowired
The staple for dependency injection, @Autowired automates the wiring of beans together in your app’s container. Essentially, it eliminates the need for getters and setters, thereby simplifying dependency management significantly.
@Controller & @RestController
@Controller and @RestController are noteworthy annotations used in Spring MVC. While @Controller perfectly combines command-based classes with model attributes, @RestController automatically adds @ResponseBody. In essence, it encapsulates your response entity and the HTTP response body in one.
@RequestMapping
@Mapping requests facilitate mapping web requests onto specific handler classes and methods. Mostly used along @Controller annotation, it guides HTTP requests to their required destinations.
@PathVariable
Quite effective in URI template scenarios, the @PathVariable annotation indicates that a method parameter is bound to a URI template variable.
@RequestBody
The @RequestBody annotation identifies an incoming request’s body and deserializes it into a Java object, as designated by the method argument.
@ResponseBody
A twin to @RequestBody, @ResponseBody, serializes the result of a method execution and throws it into an HTTP response body.
@Component, @Service, @Repository
These stereotype annotations define different components:
– @Component: General spring components
– @Service: Contains business logic
– @Repository: Handles database operations
@Qualifier
For managing confusion when multiple beans qualify for autowiring, @Qualifier proves essential. It specifies which exact bean will be wired, ensuring correct and efficient autowiring.
@Value
The @Value annotation injects values from a property file into a field. It offers a convenient way to express string-based values in beans.
@SpringBootTest
A critical annotation for writing integration tests, @SpringBootTest loads complete application context and all the beans. It’s a one-stop solution to testing in Spring Boot applications.
In summary, these annotations in Spring Boot simplify the development process significantly, reducing development time and efforts and controlling complexity within the application. However, a clear understanding of each annotation is vital to maximally leverage the power they offer in Java and Spring Boot programming.
Arming yourself with this Spring Boot annotation cheat sheet is a crucial step in your journey towards becoming a seasoned Spring Boot developer, offering you clear insights and ready-to-use tips to apply these annotations effectively.
2 thoughts on “Spring Boot Annotations Cheat Sheet”