Data Types in Python. Python, a high-level programming language, is renowned for its simplicity and readability, making it a favorite among beginners and professionals alike. A fundamental aspect of Python, and indeed all programming languages, is the concept of data types. Data types are essentially the classification of data based on the kind of value they hold or the operations that can be performed on them. This article delves into the core data types available in Python, offering insights into their characteristics and usage.
Table of Contents
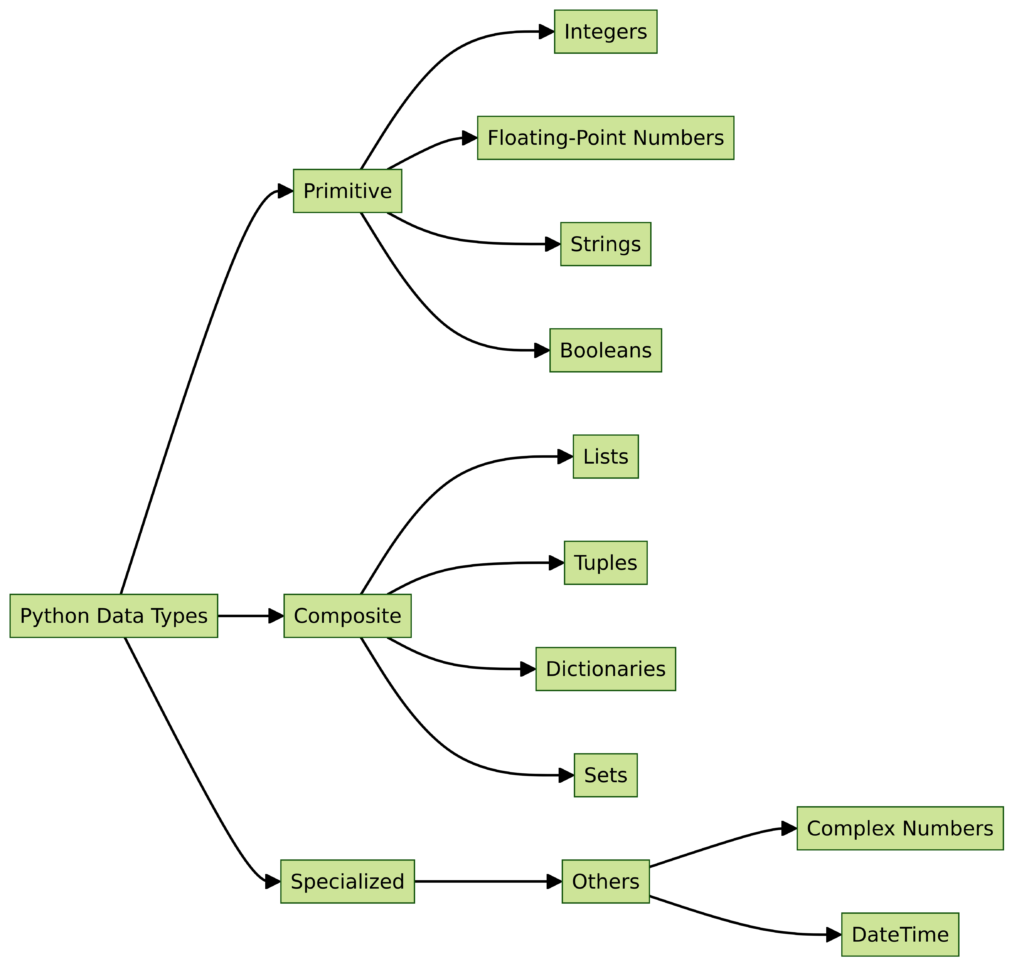
Primitive Data Types in Python
At the heart of Python’s data type system are the primitive types, which include integers, floats, strings, and booleans.
- Integers (int): These are whole numbers, positive or negative, without decimals. Examples include 42, -19, and 2020. Python 3 supports unlimited integer size, limited only by the available memory.
- Floating-Point Numbers (float): Floats represent real numbers and can include decimal points. Examples are 3.14, -0.001, and 2.0e8. They are particularly useful in mathematical computations that require precision.
- Strings: Strings are sequences of Unicode characters, enclosed in single (‘ ‘), double (” “), or triple (”’ ”’ or “”” “””) quotes. They can represent text, symbols, or numbers as text. For instance, ‘Hello, World!’, “Python3.8″, and ”’This is a multi-line string”’ are all valid strings.
- Booleans (bool): This type represents one of two values: True or False. Booleans are often the result of comparison operations and are instrumental in control flow and decision-making in programs.
Composite Data Types
Python also offers composite data types, allowing for the aggregation of primitive data types. These include lists, tuples, dictionaries, and sets.
- Lists: A list is an ordered collection of items that can be of mixed types. Lists are mutable, meaning their contents can be changed. They are defined by square brackets, with items separated by commas, e.g.,
[1, 'two', 3.0]
. - Tuples: Similar to lists, tuples are ordered collections of items. However, tuples are immutable; once created, their contents cannot be altered. Tuples are defined by parentheses, as in
(1, 'two', 3.0)
. - Dictionaries: Dictionaries are unordered collections of key-value pairs. They are mutable and indexed by unique keys, making them ideal for representing complex data structures. Dictionaries are defined with curly braces,
{}
. An example is{'name': 'Alice', 'age': 30}
. - Sets: A set is an unordered collection of unique items. Sets are mutable and useful for operations involving membership testing, removing duplicates, and mathematical operations like unions and intersections. They are defined with curly braces, e.g.,
{1, 2, 3, 4}
.
Specialized Data Types
Beyond these core types, Python supports several specialized data types, including complex numbers for mathematical computations, which are represented as complex(real, imag)
, and various data types defined in modules such as datetime
for date and time manipulation.
Choosing the Right Data Type
Selecting the appropriate data type is crucial for efficient programming. The choice depends on the nature of the data and the intended operations. For example, use lists for ordered collections of items that might change over time, dictionaries for associating related information with key lookup, and sets for handling unique elements.
Understanding data types in Python is fundamental to mastering the language. Each data type serves specific purposes and comes with its operations and methods. By grasitating the nuances of Python’s data types, developers can write more efficient, readable, and robust code. As you continue your Python journey, remember to experiment with these data types, explore their methods, and learn how they interact with each other in various scenarios.