The Go Programming Language, also known as Golang, is a statically typed, compiled programming language that has gained immense popularity for it’s simplicity, efficiency, and built-in concurrency support. In this article we are going to explore about golang for loop and it’s various uses.
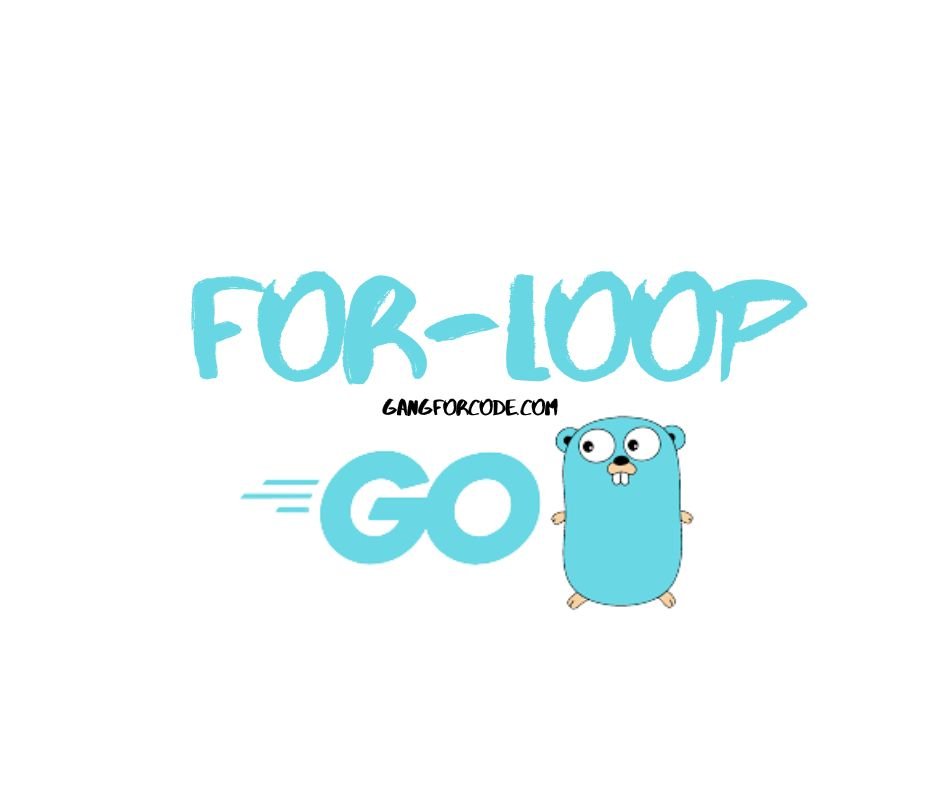
Golang for Loop Basics
The general & basic syntax of a Golang for loop is as follows –
for initialization; condition; update {
// Code to be executed repeatedly
}
- Initialization: This part is executed only once at the start of loop and is typically used to initialize loop control variables.
- Condition: The loop continues executing as long as this condition is true. Once the condition becomes false, the loop exits.
- Update: This is usually an increment or decrement operation that modifies the loop control variable, moving towards the condition becoming false.
A simple for-loop example that prints numbers from 1 to 10 is as follows –
// Simple for loop to print numbers from 1 to 10
for i := 1; i <= 10; i++ {
fmt.Println(i)
}
Golang for loop range
The Golang for loop range
keyword allows us to iterate over the elements of a slice, array, map, or string. Here The range
keyword returns two values: the index and the value. An example of golang for loop with range keyword is given bellow
package main
import "fmt"
func main() {
numbers := []int{1, 3, 4, 5, 6, 7, 34, 65}
for index, value := range numbers {
fmt.Printf("Index: %d, Value: %d\n", index, value)
}
}

Golang for Loop Array
Arrays are a fundamental data structure in Golang, and we can easily iterate Arrays with for loop in Golang and perform various operation with Arrays.
package main
import "fmt"
func main() {
numbers := [5]int{2, 4, 6, 8, 10}
sum := 0
for _, value := range numbers {
sum += value
}
fmt.Printf("Sum of elements: %d\n", sum)
}

1 thought on “Golang for Loop – Let’s Learn”