In this tutorial, we are going to write a C program that calculates the circumference of a circle.
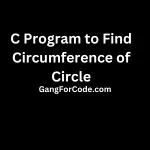
Formula for Circumference of a Circle
The circumference of a Circle is the total length around its boundary. It is calculated by using the formula
Circumference = 2*π*r
Where
- π is a mathematical constant approximately equal to 3.14159
- r is the radius of the circle.
C Program for Circumference of Circle
#include <stdio.h>
#define PI 3.14159 // Defining the value of Pi
float calculateCircumference(float radius)
{
return 2 * PI * radius;
}
void main()
{
float radius;
printf("Enter the radius");
scanf("%f", &radius);
if (radius < 0)
{
printf("Radius cannot be zero");
}
else
{
printf("Circumference of given circle = %f", calculateCircumference(radius));
}
}
Output-

How the Above C Program Work?
- The program starts by asking the user to input the radius of the circle.
- In the program, we define the mathematical constant π by using
# to define
the preprocessor directive. - The calculateCircumference function takes the circle’s radius as argumnet and returns the circumference using the abovementioned formula.
- In the main function after taking input from the user it takes if the radius is non-negative.
- Finally, the program calculates and displays the circumference of the circle based on the provided radius.
4 thoughts on “C Program to Find Circumference of Circle”