In this article, we are going to write a C program to search for an element in the given array.
Table of Contents
Objective
The objective of this C program is to search for a given element within an array. This will involve iterating through the elements of the array and comparing each element with the target value to determine if a given element exists within an array or not.
C Program to Search an Element
Let’s write a C program that searchhes for a given elment within an array.
#include <stdio.h>
void main()
{
int n, target, flag = 0;
int array[100];
printf("Enter the size of array");
scanf("%d", &n);
printf("Enter elements of array one by one \n");
for (int i = 0; i < n; i++)
{
scanf("%d", &array[i]);
}
printf("Enter the element to search");
scanf("%d", &target);
for (int i = 0; i < n; i++)
{
if (array[i] == target)
{
flag = 1;
break;
}
}
if (flag)
{
printf("Element is present in the array");
}
else
{
printf("Element is not present in the array");
}
}
Output
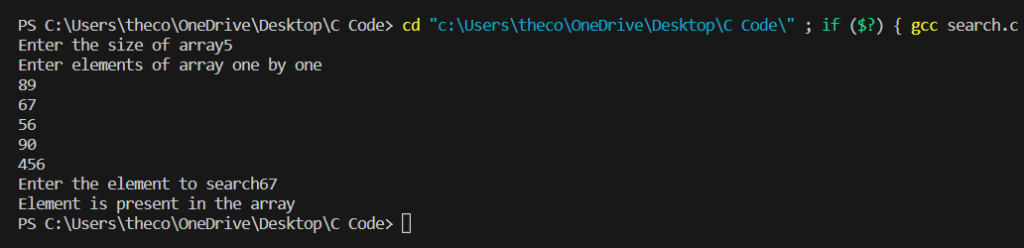
The above C program asks the user to input the size of the array and its elements. after that ask the user to enter the elements to be searched. After that using a loop, it iterates through the array element and compares each element with the the target value. If the element is found it updates the flag value to 1. If flag==1 then it prints “Element is present in the array” else it prints “Element is not present in the array”.