Ensuring a directory is empty can be a crucial check in many Python applications, whether you’re cleaning up data, verifying configurations, or setting up environments. Python, with its comprehensive standard library, offers straightforward methods to perform this task. In this tutorial, we’ll explore a concise yet efficient approach to determine if a directory is empty using Python.
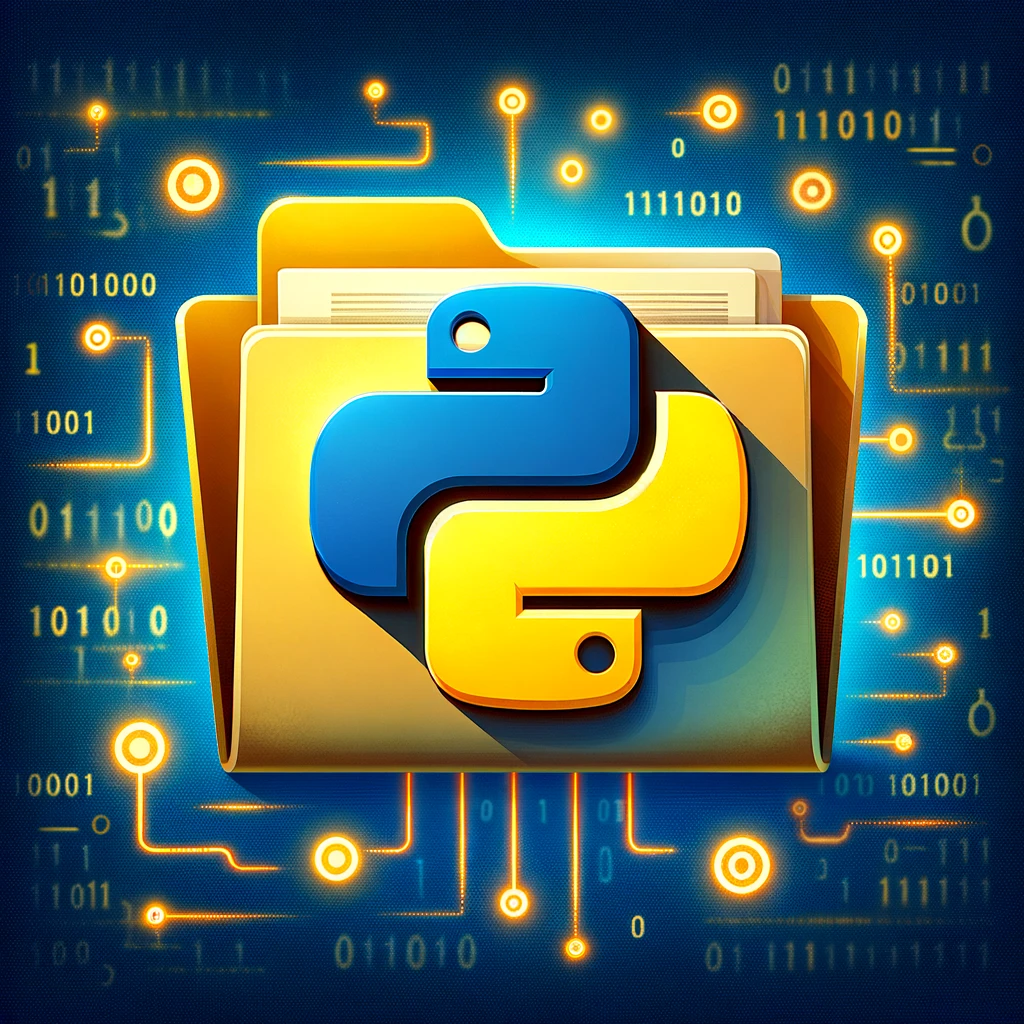
Table of Contents
How to Check If a Directory is Empty
To check if a directory is empty, you’ll primarily need to use the os
module, which provides a portable way of using operating system-dependent functionality. The method we’ll focus on involves listing the contents of the directory and then checking the length of this list.
Python Code for checking Empty Directory
import os
# directory path
directory_path = "C:/Users/Author/Desktop/Python"
if not os.listdir(directory_path):
print("The directory is empty.")
else:
print("The directory is not empty.")
Output

Step-by-Step Guide
Step 1: Importing the Required Module
First, you need to import the os
module, which will allow you to interact with the operating system:
import os
Step 2: Define the Directory Path
Specify the path to the directory you want to check. Replace 'your_directory_path'
with the actual path of your directory:
directory_path = 'your_directory_path'
Step 3: Checking if the Directory is Empty
Use the os.listdir()
function to get a list of everything in the directory specified by directory_path
. Then, check if this list is empty:
if not os.listdir(directory_path):
print("The directory is empty.")
else:
print("The directory is not empty.")
The os.listdir(directory_path)
function returns a list of the names of the entries in the directory given by path. The condition if not os.listdir(directory_path)
evaluates to True
if this list is empty, indicating the directory is empty.
Checking if a directory is empty is a common task that can be easily accomplished in Python using the os
module. This tutorial provided a simple and efficient method to perform this check, suitable for various applications and workflows. Remember, Python’s standard library is rich with functionality that can help you interact with the operating system, making tasks like these straightforward to implement.
Happy Coding & Learning