In this article we are going to claculate factorial of a number using C Program. You can check more C Programming tutorial in C section .
Factorial in C
Steps for Calculating Factorial in C
- Create a variable result and initialize by 1 because factorial of 0 is 1 (factorial base condition)
- take a non negative input from user to calculate Factorial using Scanf()
- run a for loop from i=1 to i <= number
- perform result = result*i
- End of loop
- print the result
- Exit
Factorial Program in C
#include<stdio.h>
void main ()
{
int number, i, result = 1;
printf ("enter the number\n");
scanf ("%d", &number);
if (number >= 0)
{
for (i = 1; i <= number; i++)
{
result = result * i;
}
printf ("factorial of %d = %d", number, result);
}
else
printf (" Wrong Input");
}
Factorial Program in C output
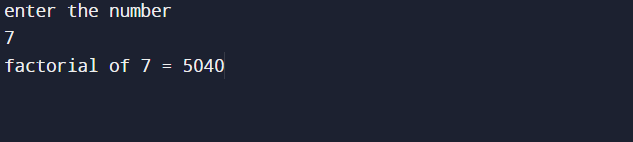
Similar C Tutorials
Sum and Average in C – https://gangforcode.com/c-program-for-taking-input-of-marks-and-calculating-sum-and-average/
Sum of odd and even numbers in C – https://gangforcode.com/c-program-to-print-sum-of-even-and-odd-numbers/