FCFS Scheduling Program in Python. First Come, First Served (FCFS) is one of the simplest and most Straightforward CPU Scheduling Algorithms. As the name suggests, the algorithm schedules the processes in the order they arrived in the ready queue. This article will walk you through creating an FCFS scheduling Program in Python.
Table of Contents
FCFS Scheduling Algorithm Steps
The steps for the FCFS scheduling algorithm are as follows:
- Input the process along with their burst times.
- Sort the Processes by their arrival time.
- Compute the start and finish times for each process.
- Calculate the waiting time and turnaround time for each process.
- Compute the average waiting time and turnaround time.
FCFS Python Implementation
Here is a Python implementation of the FCFS Scheduling Algorithm:
Defining the Process Class
Let’s define a class that represents a process. Each process will have attributes like Process ID, arrival time, and burst time.
# Class to represent a process
class Process:
def __init__(self, pid, arrival_time, burst_time):
self.pid = pid
self.arrival_time = arrival_time
self.burst_time = burst_time
self.start_time = 0
self.finish_time = 0
self.waiting_time = 0
self.turnaround_time = 0
def fcfs_scheduling(processes):
# Sort processes by arrival time
processes.sort(key=lambda x: x.arrival_time)
current_time = 0
for process in processes:
if current_time < process.arrival_time:
current_time = process.arrival_time
process.start_time = current_time
process.finish_time = process.start_time + process.burst_time
process.waiting_time = process.start_time - process.arrival_time
process.turnaround_time = process.finish_time - process.arrival_time
current_time += process.burst_time
return processes
def print_processes(processes):
print("PID\tArrival\tBurst\tStart\tFinish\tWaiting\tTurnaround")
for process in processes:
print(f"{process.pid}\t{process.arrival_time}\t{process.burst_time}\t"
f"{process.start_time}\t{process.finish_time}\t{process.waiting_time}\t"
f"{process.turnaround_time}")
def main():
processes = [
Process(1, 0, 4),
Process(2, 1, 3),
Process(3, 2, 1),
Process(4, 3, 2)
]
processes = fcfs_scheduling(processes)
print_processes(processes)
if __name__ == "__main__":
main()
Example and Explanation
Let’s break down the example provided in the Above Python code
- Process initialization: We created a list of processes with their respective arrival and burst times.
- Scheduling: The
fcfs_scheduling
function sorts the processes by their arrival time and calculates the start time, finish time, waiting time, and turnaround time for each process. - Output: The
print_processes
function prints the details of each process.
Output
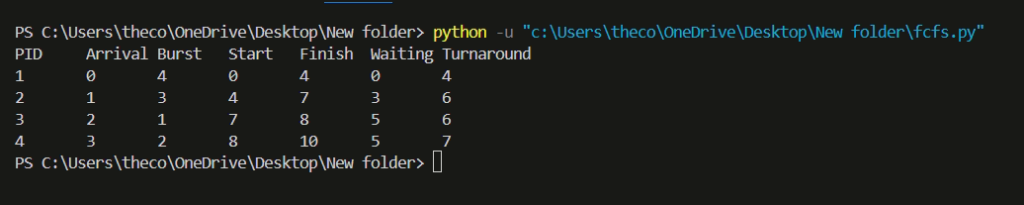
Explanation
- Process 1 arrives at time 0 and starts immediately, finishing at time 4.
- Process 2 arrives at time 1 but wait until process 1 finishes. It starts at time 4 and finishes at time 7.
- Process 3 arrives at time 2 and waits for processes 1 and 2 to finish, starting at time 7 and finishing at time 8.
- Process 4 arrives at time 4 and waits for all previous processes to finish, starting at time 8 and finishing at time 10.
The FCFS scheduling algorithm is simple to implement but may not always be efficient due to potential long waiting times for shorter processes.
Happy Coding and Learning