In modern web applications, storing images in databases is a common practice, allowing for centralized data management and retrieval. In this tutorial, we are going to store images in a MySQL database using Spring Boot.

Table of Contents
Prerequisites
Before we start, ensure you have the following prerequisites:
- Java Development Kit (JDK) installed on your machine.
- MySQL database server installed locally or remotely with appropriate access credentials.
- An IDE for development
Setting Up the Spring Boot Project
Create a Spring Boot Project
Let’s create our spring boot project. You can use https://start.spring.io/ for creating spring boot projects. Make sure that you have included all the necessary dependencies like Spring Web, Spring Data JPA, and MySQL Driver in your project.
MySQL Properties for Spring Boot
Now we have to Configure application.properties
or application.yml
file to set up the MySQL database connection. Replace the username and password with your MySQL database credentials
application.properties
# Database Configuration
spring.datasource.url=jdbc:mysql://localhost:3306/mysql-image?createDatabaseIfNotExist=true
spring.datasource.username=root
spring.datasource.password=root@123
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
# Hibernate properties
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQLDialect
spring.jpa.hibernate.ddl-auto=update
Entity Class for Storing Images
Let’s create an entity class to represent the image entity and map it to the database using JPA annotations. Here’s our entity class names FileObj
.
FileObj.Java
package com.gangforcode.mysqlimage.entity;
import jakarta.persistence.*;
@Entity
public class FileObj {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String contentType;
@Lob
@Column(columnDefinition = "LONGBLOB")
private byte[] data;
public FileObj() {
}
public FileObj(Long id, String name, String contentType, byte[] data) {
this.id = id;
this.name = name;
this.contentType = contentType;
this.data = data;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getContentType() {
return contentType;
}
public void setContentType(String contentType) {
this.contentType = contentType;
}
public byte[] getData() {
return data;
}
public void setData(byte[] data) {
this.data = data;
}
}
JPA Repository
Let’s create a repository interface that extends JpaRepository
to handle CRUD operations for the FileObj
.
FileRepo.java
package com.gangforcode.mysqlimage.repo;
import com.gangforcode.mysqlimage.entity.FileObj;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface FileRepo extends JpaRepository<FileObj,Long>{
}
Service Layer Implementation
Let’s implement our Service class for business logic. Here we are creating two methods one for storing images to MySQL and one for fetching images from MySQL Database.
ImageService.java
package com.gangforcode.mysqlimage.service;
import com.gangforcode.mysqlimage.entity.FileObj;
import com.gangforcode.mysqlimage.repo.FileRepo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
@Service
public class ImageService {
@Autowired
private FileRepo imageRepository;
public FileObj saveImage(MultipartFile file) throws IOException {
FileObj fileObj = new FileObj() ;
fileObj.setName(file.getOriginalFilename());
fileObj.setContentType(file.getContentType());
fileObj.setData(file.getBytes());
return imageRepository.save(fileObj);
}
public FileObj findById(Long id) throws Exception {
FileObj image = imageRepository.findById(id).orElseThrow(() -> new Exception("Image not found"));
return image;
}
}
Controller Implementation
Let’s Create our controller. The ImageController
is a class in our Spring Boot application that handles incoming HTTP requests related to image operations. It serves as an interface between the client (e.g., web browser, mobile app) and the backend logic responsible for managing image storage, retrieval, and other related functionalities
ImageController.java
package com.gangforcode.mysqlimage;
import com.gangforcode.mysqlimage.entity.FileObj;
import com.gangforcode.mysqlimage.service.ImageService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
@RestController
@RequestMapping("/api/images")
public class ImageController {
@Autowired
private ImageService imageService;
@PostMapping
public ResponseEntity<FileObj> uploadImage(@RequestParam("file") MultipartFile file) throws IOException {
return ResponseEntity.ok(imageService.saveImage(file));
}
@GetMapping("/{id}")
public ResponseEntity<byte[]> getImage(@PathVariable Long id) throws Exception {
FileObj image = imageService.findById(id);
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.parseMediaType(image.getContentType()));
return ResponseEntity.ok()
.headers(headers)
.body(image.getData());
}
}
Testing the End-Points
Our Spring Boot Application is ready let’s run this application and test in Post-Man
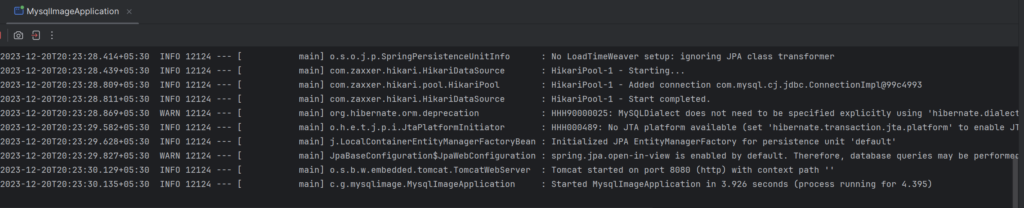
Testing end points in Post-Man
Storing Images in MySQL
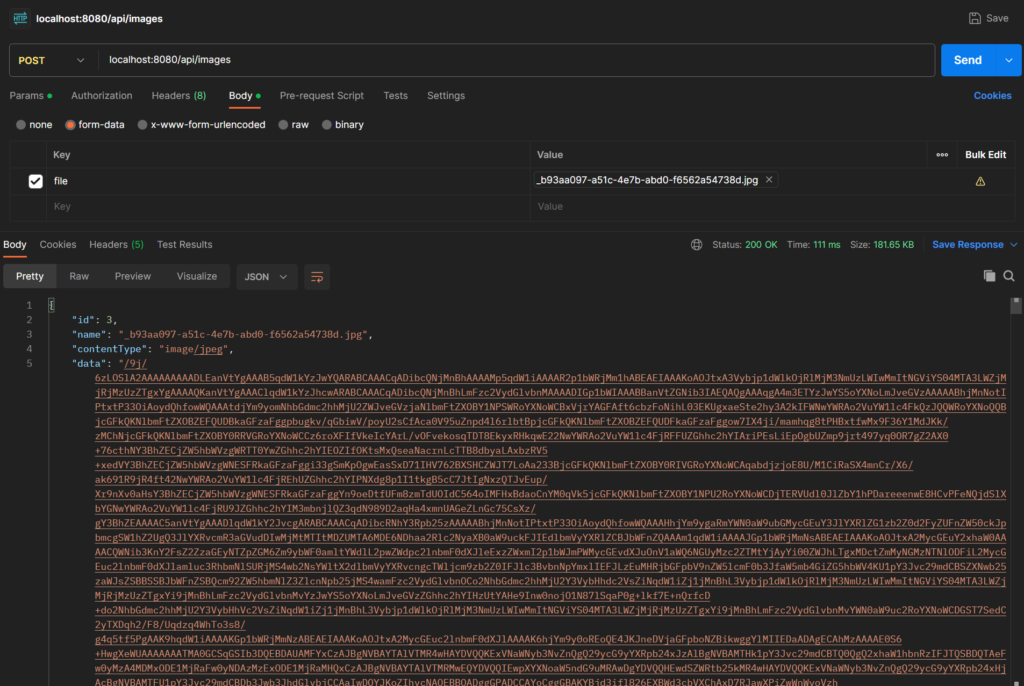
Getting Images from MySQL
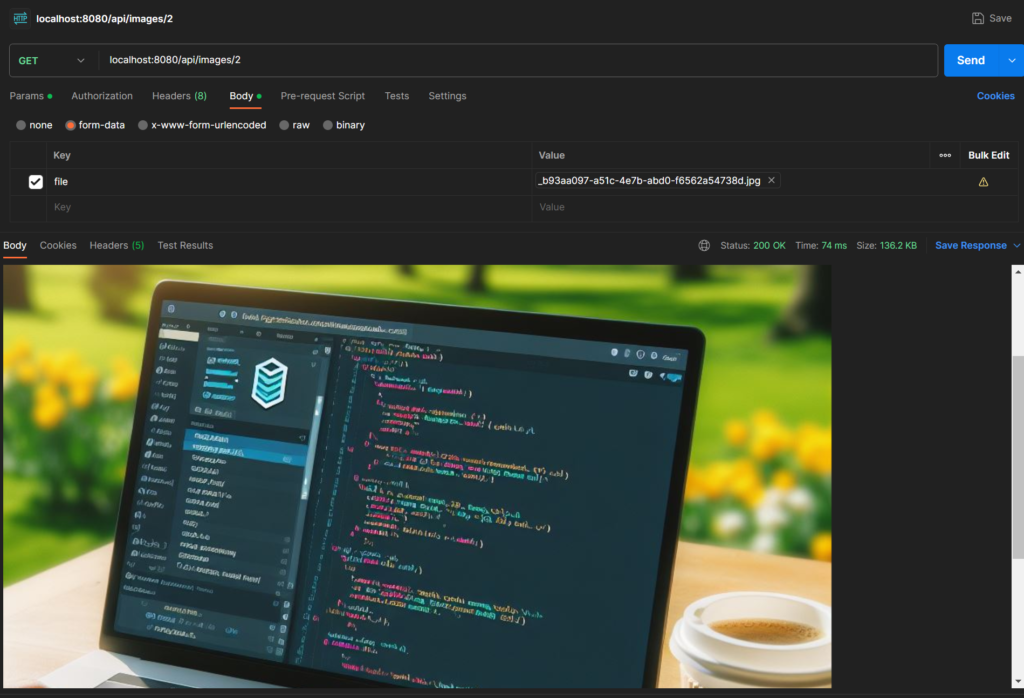
That’s all for this tutorial, the entire source code is available here. Congratulations! You’ve learned how to store images in a MySQL database using Spring Boot. This tutorial provides a basic setup, and you can extend this functionality for more advanced features like image resizing, validation, or implementing additional endpoints for image manipulation. Always consider best practices and security measures when handling file uploads in a production environment.
Thanks !!!
Really helpfull