In this article we are going to create a C program to compute the area of a rectangle using functions, demonstrating the modular and organized nature of C programming.
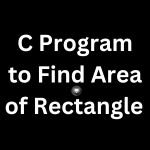
Understanding the Problem
The area of a rectangle is calculated by multiplying its length and width. To compute this in C, we will create a program that takes input for the length and width of the rectangle from the user and calculates its area using a separate function.
Writing the C Program to find Area of Rectangle Using Function
#include <stdio.h>
// Function to calculate the area of a rectangle
float calculateArea(float length, float width) {
return length * width;
}
void main() {
float length, width;
// Taking user input for length and width of the rectangle
printf("Enter the length of the rectangle: ");
scanf("%f", &length);
printf("Enter the width of the rectangle: ");
scanf("%f", &width);
// Calling the function to calculate area and storing the result
float area = calculateArea(length, width);
// Displaying the calculated area
printf("The area of the rectangle is: %.2f\n", area);
}
Output

Explanation of the Program
- Header Files: The
#include <stdio.h>
statement is included for input/output operations. calculateArea
Function: This function takes two arguments—length
andwidth
—and returns the product of these values, which represents the area of the rectangle.main()
Function:- Declares variables
length
andwidth
to store user-input values. - Prompts the user to input the length and width of the rectangle using
printf()
andscanf()
functions. - Calls the
calculateArea()
function with user-provided length and width as arguments and stores the result in thearea
variable. - Displays the calculated area using
printf()
.
- Declares variables
Creating a C program to find the area of a rectangle using functions showcases the organization and modular approach of C programming. Functions allow us to encapsulate specific tasks, promoting reusability and readability of the code. Understanding these fundamentals of C programming forms a solid foundation for tackling more complex problems efficiently.
3 thoughts on “C Program to Find Area of Rectangle Using Function ”