Email Validation in React JS. Email validation is a crucial aspect of modern web applications, ensuring that user input is correct and functional. For developers using React JS, implementing robust email validation can enhance form handling and improve user interactions. This article provides a comprehensive guide on how to validate email addresses in your React applications effectively.
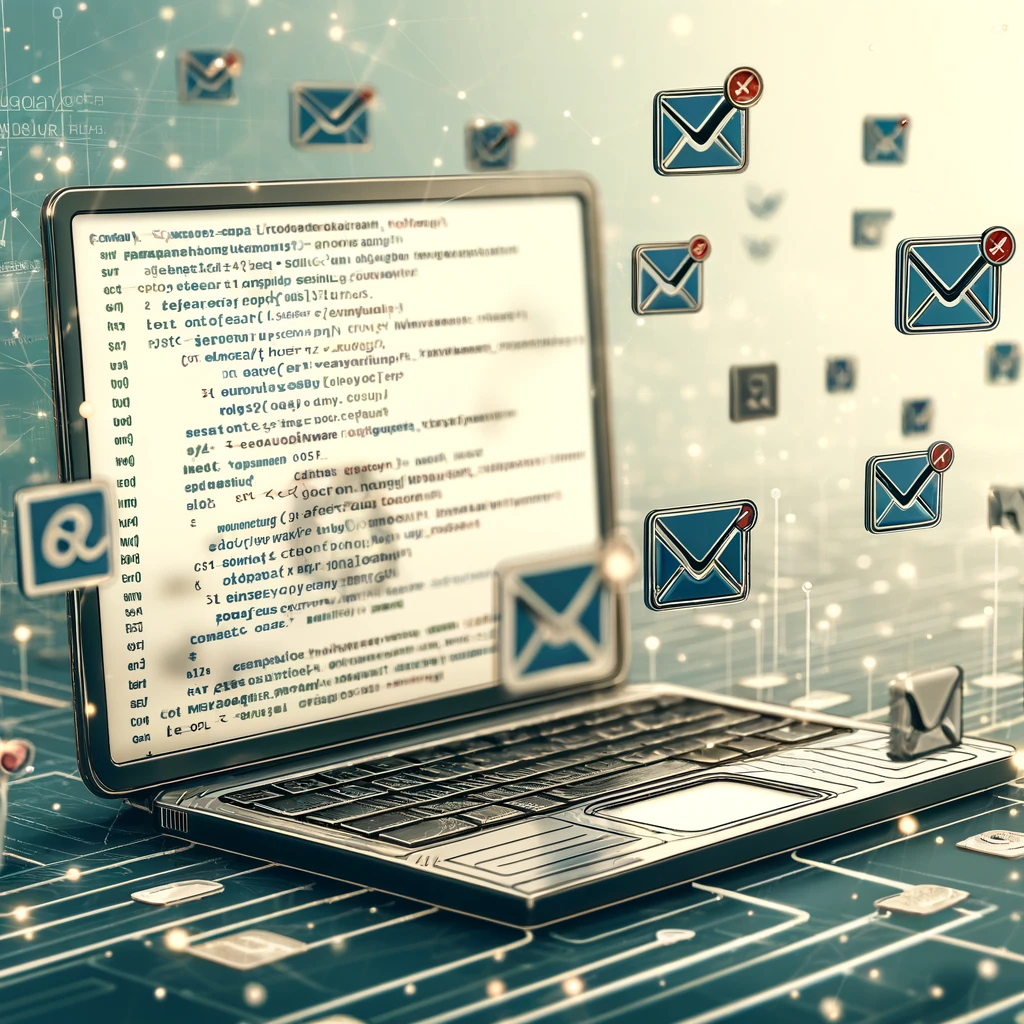
Table of Contents
Methods of Email Validation in React JS
1. Using HTML5 Input Types
The simplest way to start validating emails is by using the HTML5 input type email
. This method uses browser capabilities to check the basic syntax of an email:
<input type="email" name="userEmail" required />
While this approach is easy to implement, it only checks if the email format is correct (like including an “@” symbol and a domain), and does not validate whether the email domain itself can receive emails.
2. Regular Expression (Regex) Matching
For a more thorough validation, you can use regular expressions to match against the email string. Here’s a basic example of how to implement this in a React component:
function validateEmail(email) {
const regex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return regex.test(email);
}
function EmailInputComponent() {
const [email, setEmail] = React.useState("");
const handleInputChange = (e) => {
const { value } = e.target;
setEmail(value);
if (validateEmail(value)) {
console.log("Email is valid");
} else {
console.log("Invalid email");
}
};
return <input type="text" value={email} onChange={handleInputChange} />;
}
This method provides more control over what is considered a valid email but requires maintaining regex patterns, which can become complex and hard to manage for nuanced validations.
3. Using External Libraries for Email Validation
For developers who prefer not to reinvent the wheel, several libraries can help with email validation. One popular choice is validator
, a library that provides a variety of string validation methods, including email validation:
npm install validator
import validator from 'validator';
function EmailInputComponent() {
const [email, setEmail] = React.useState("");
const handleInputChange = (e) => {
const { value } = e.target;
setEmail(value);
if (validator.isEmail(value)) {
console.log("Email is valid");
} else {
console.log("Invalid email");
}
};
return <input type="text" value={email} onChange={handleInputChange} />;
}
This method is easy to implement, reduces the complexity of regex patterns, and provides robust validation techniques.
Why Validate Emails in React JS?
React JS offers a structured approach to building user interfaces with components. Validating emails within these components helps maintain a clean and manageable codebase, ensures data validation at the UI level, and provides immediate feedback to users, enhancing the overall user experience.
Best Practices
- Immediate Feedback: Provide real-time validation feedback to users as they type. This helps catch errors early and improves user satisfaction.
- Accessibility: Ensure that error messages and form labels are accessible to all users, including those using screen readers.
- Security: Always validate email inputs on the server side as well to prevent malicious data from entering your system.
Email validation is a critical component of modern web applications. React JS developers have multiple methods at their disposal to ensure robust validation. Whether you choose the simplicity of HTML5, the control of regex, or the convenience of an external library, your focus should be on creating a seamless and secure user experience. By following best practices and using the appropriate tools, you can efficiently implement effective email validation in your React applications.
Happy Coding & Learning
See Also