Factorial calculation is a fundamental mathematical operation frequently used in various domains such as mathematics, computer science, and engineering. In Python, factorial calculation can be implemented using various methods, one of which involves recursion.
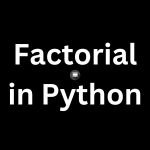
Recursion is a powerful programming technique where a function calls itself to solve a smaller instance of the same problem until it reaches a base case.
Factorial in Python Using Recursion
Let’s delve into a Python program that computes the factorial of a number using recursion:
def factorial(n):
if n == 0 or n == 1: # Base case: factorial of 0 or 1 is 1
return 1
else:
return n * factorial(n - 1) # Recursive call
# Taking input from user
n = int(input("Enter the number "))
# Checking for negative input
if n < 0:
print("Invalid Input")
else:
print(f"The factorial of {n} is {factorial(n)}")
Output

The above Python program demonstrates the use of a recursive function called factorial
. The function takes an integer n
as input and calculates its factorial using recursion.
Understanding Python Factorial Program Using Recursion
- The
factorial
function takes an argumentn
, representing the number whose factorial needs to be calculated. - It checks for the base case: if
n
is 0 or 1, it returns 1 because the factorial of 0 and 1 is 1. - For any other positive integer
n
, the function computes the factorial by multiplyingn
with the factorial of(n - 1)
, thereby reducing the problem size and utilizing recursion to solve smaller instances of the same problem. - The user is prompted to input a non-negative integer. If the input is negative, the program informs the user that factorial is not defined for negative numbers. Otherwise, it calculates the factorial of the input number using the
factorial
function and displays the result.
Recursion provides an elegant way to solve problems by breaking them down into smaller, more manageable subproblems. However, it’s essential to be cautious with recursion as it can lead to stack overflow errors for significantly large inputs due to excessive function calls.
2 thoughts on “Python Factorial Program Using Recursion”