Spring Boot has become the go-to choice for developers when it comes to building robust and scalable applications. MongoDB, on the other hand, is a NoSQL database known for its flexibility and scalability. Combining Spring Boot’s power with MongoDB we can create efficient and high-performing applications.
In this article, we are going to show you how to perform CRUD (Create, Read, Update, Delete) operations on a MongoDB database using Spring Boot.
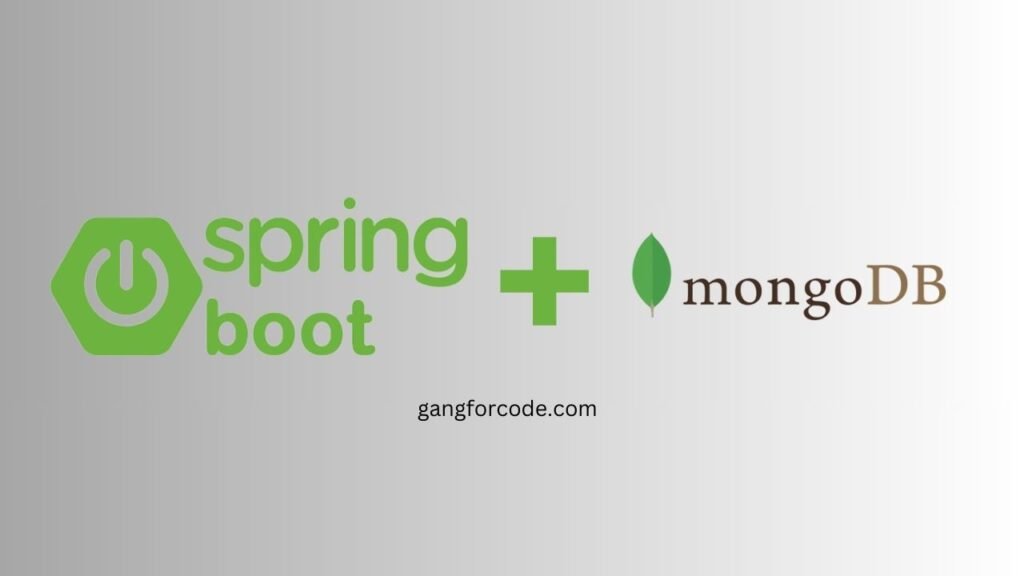
Table of Contents
Prerequisites
To Perform Spring Boot CRUD Operations with MongoDB, you will need the following:
- A MongoDB database running locally
- A Java IDE, such as IntelliJ IDEA
Creating Spring Boot Project
To get started, first we need a Spring Boot Project with required dependencies. To create a new Spring Boot project use your preferred IDE or the Spring Initializer (https://start.spring.io/). Make sure to include the necessary dependencies such as ‘Spring Web’ and ‘Spring Data MongoDB.’
Spring Web and Spring Data MongoDB dependencies
We can also add Dependencies manually in the pom.xml
file. Add following dependencies in your Spring Boot Project
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
Spring Boot MongoDB Setup
Now we have to configure the MongoDB connection in the 'application.properties
‘ or 'application.yml'
file. Here we have to provide the MongoDB server URI and database name.
application.properties
spring.data.mongodb.uri=mongodb://localhost:27017/mongodb-crud
The spring.data.mongodb.uri
property specifies the connection string to the MongoDB database server. In this case, the MongoDB database is running on localhost on port 27017 and the database name is mongodb-crud
.
Data Model
Let’s create our data model class for our application. Here we are creating User Class. This class will represent the document in MongoDB Database.
User.java
package com.gangforcode.mongodbcrud.model;
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.mapping.Document;
@Document
public class User {
@Id
private String userId;
private String userName;
private String name;
public String getUserId() {
return userId;
}
public void setUserId(String userId) {
this.userId = userId;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public User(String userId, String userName, String name) {
this.userId = userId;
this.userName = userName;
this.name = name;
}
}
Repository
Let’s create the Repository interface by extending MongoRepository
. Repository is an interface that provides access to the data in MongoDB database. Spring Data MongoDB provides a number of repository interfaces that we can use, like MongoRepository
,CrudRepository
and PagingAndSortingRepository
.
UserRepository.java
package com.gangforcode.mongodbcrud.repo;
import com.gangforcode.mongodbcrud.model.User;
import org.springframework.data.mongodb.repository.MongoRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface UserRepository extends MongoRepository<User,String> {
}
Service Layer
Let’s create our service class, and perform crud operations. Inside this service class we autowired the Repository interface.
@Autowired
private UserRepository repository;
Let’s Implement CRUD Operations
Add User / Create User
To add / Create a new user to the MongoDB collection, use the save()
method provided by the repository
public User addUser(User user) {
return repository.save(user);
}
Read User / Get User
Get All Users
For getting all users from database we can use findAll()
method provided by repository
public List<User> getAllUsers() {
return repository.findAll();
}
Get User by Id
For getting a single User from database we can use findById()
method provided by repository
public Optional<User> getUserById(String Id){
return repository.findById(Id);
}
Update User
To update an user’s information, first we have to retrieve the user by ID and then modify the fields we want to change. Finally, use the save()
method to update the document
public User updateUser(String Id,User user){
User existingUser = repository.findById(Id).orElse(null);
if(existingUser!=null){
existingUser.setUserName(user.getUserName());
existingUser.setName(user.getName());
return repository.save(existingUser);
}
return null;
}
Delete User
To delete an user from the collection, we have to use the deleteById()
method:
public void deleteById(String Id){
repository.deleteById(Id);
}
Now our service Class implementation is competed with all crud operations methods
UserService.java
package com.gangforcode.mongodbcrud.service;
import com.gangforcode.mongodbcrud.model.User;
import com.gangforcode.mongodbcrud.repo.UserRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
import java.util.Optional;
@Service
public class UserService {
@Autowired
private UserRepository repository;
public User addUser(User user) {
return repository.save(user);
}
public List<User> getAllUsers() {
return repository.findAll();
}
public Optional<User> getUserById(String Id){
return repository.findById(Id);
}
public User updateUser(String Id,User user){
User existingUser = repository.findById(Id).orElse(null);
if(existingUser!=null){
existingUser.setUserName(user.getUserName());
existingUser.setName(user.getName());
return repository.save(existingUser);
}
return null;
}
public void deleteById(String Id){
repository.deleteById(Id);
}
}
Controller
The controller is responsible for handling HTTP requests of application. It will use the service Class to perform CRUD operations on the data model.
UserController.java
package com.gangforcode.mongodbcrud.controller;
import com.gangforcode.mongodbcrud.model.User;
import com.gangforcode.mongodbcrud.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
import java.util.Optional;
@RestController
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService service;
// adding new user
@PostMapping("/add")
public User addUser(@RequestBody User user){
return service.addUser(user);
}
// update user info
@PutMapping("/update/{id}")
public User updateUser(@PathVariable String id, @RequestBody User user) {
return service.updateUser(id,user);
}
// Get all users
@GetMapping("/getAll")
public List<User> getAllUsers(){
return service.getAllUsers();
}
// Get user by id
@GetMapping("/getById/{id}")
public Optional<User> getUserById(@PathVariable String id){
return service.getUserById(id);
}
// Delete user by id
@DeleteMapping("/delete/{id}")
public void deleteUser(@PathVariable String id){
service.deleteById(id);
}
}
Now we can run the spring boot application and test all end points. You can download this entire project https://github.com/thecodedata/mongodb-springboot-crud
1 thought on “Spring Boot CRUD Operations with MongoDB”