In the world of C programming, pointers stand as powerful entities that facilitate direct memory manipulation. Pointers hold memory addresses and allow us to access and modify data indirectly, offering flexibility and efficiency in programming. Let’s write a simple C program that swaps the values of two numbers using pointers
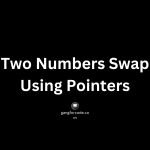
C Pointer Basics
A pointer in C is a variable that holds the memory address of another variable. By dereferencing a pointer, we can access or modify the value stored at that address. This functionality forms the core of various operations, including swapping two variables without using a temporary variable.
C Program to Swap Two Numbers Using Pointers
// C Program to Swap Two Numbers Using Pointers
#include <stdio.h>
// Function to swap two numbers using pointers
void swapNumbers(int *ptr1, int *ptr2) {
*ptr1 = *ptr1 + *ptr2;
*ptr2 = *ptr1 - *ptr2;
*ptr1 = *ptr1 - *ptr2;
}
void main() {
int num1, num2;
// Input for two numbers
printf("Enter first number: ");
scanf("%d", &num1);
printf("Enter second number: ");
scanf("%d", &num2);
// Displaying original numbers
printf("\nOriginal numbers: num 1 = %d, num 2 = %d\n", num1, num2);
// Calling the function to swap numbers using pointers
swapNumbers(&num1, &num2);
// Displaying swapped numbers
printf("Swapped numbers: num 1 = %d, num 2 = %d\n", num1, num2);
}
Output

C Program to Swap Two Numbers Using Pointers – Explanation
swapNumbers
Function:- This function takes two integer pointers
ptr1
andptr2
as parameters. - Using pointer dereferencing (
*ptr1
and*ptr2
), it performs arithmetic operations to swap the values of the numbers without using a temporary variable.
- This function takes two integer pointers
main()
Function:- Declares variables
num1
andnum2
to store user-input values. - Prompts the user to input two numbers.
- Displays the original numbers using
printf()
. - Calls the
swapNumbers()
function by passing the addresses ofnum1
andnum2
. - Displays the swapped numbers using
printf()
.
- Declares variables
5 thoughts on “C Program to Swap Two Numbers Using Pointers”